|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
EDX 数据寄存器,常用于数据传递。
pushl %ebp 保存调用函数的基址到栈中,
movl %esp, %ebp 设置EBP为当前被调用函数的基址指针,即当前栈顶
subl $xx, %esp 为当前函数分配xx字节栈空间用于存储局部变量
movl %ebp, %esp 释放被调函数栈空间
popl %ebp 恢复ebp为调用函数基址
int ArraySum(int *array, int n){
int t = 0;
for(int i=0; i<n; ++i) t += array[i];
return t;
}
int main() {
int a[5] = {1, 2, 3, 4, 5 };
int sum = ArraySum(a, 5);
return sum;
}
gcc -std=c99 -S -o sum.s sum.c
ArraySum:
pushl %ebp
movl %esp, %ebp
subl $16, %esp //分配16字节栈空间
movl $0, -8(%ebp) //初始化t
movl $0, -4(%ebp) //初始化i
jmp .L2
.L3:
movl -4(%ebp), %eax
sall $2, %eax //i<<2, 即i*4, 一个int占4字节
addl 8(%ebp), %eax //得到array[i]地址,array+i*4
movl (%eax), %eax //array[i]
addl %eax, -8(%ebp) //t+=array[i]
addl $1, -4(%ebp)
.L2:
movl -4(%ebp), %eax
cmpl 12(%ebp), %eax //比较i<n
jl .L3
movl -8(%ebp), %eax //return t; 默认eax存函数返回值
leave
ret
main:
.LFB1:
pushl %ebp
movl %esp, %ebp
subl $40, %esp
movl $1, -24(%ebp) //初始化a[0]
movl $2, -20(%ebp) //初始化a[1]
movl $3, -16(%ebp) //初始化a[2]
movl $4, -12(%ebp) //初始化a[3]
movl $5, -8(%ebp) //初始化a[4]
movl $5, 4(%esp) //5作为第二个参数传给 ArraySum
leal -24(%ebp), %eax //leal产生数组a的地址
movl %eax, (%esp) //作为第一个参数传给ArraySum
call ArraySum
movl %eax, -4(%ebp) //返回值传给sum
movl -4(%ebp), %eax //return sum
leave
ret
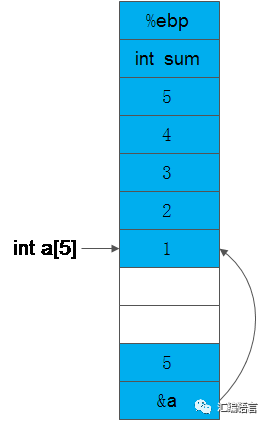
struct Point{
int x;
int y;
};
void PointInit(struct Point *p, int x, int y){
p->x = x;
p->y = y;
}
int main() {
struct Point p;
int x = 10;
int y = 20;
PointInit(&p, x, y);
return 0;
}
PointInit:
pushl %ebp
movl %esp, %ebp
movl 8(%ebp), %eax //p的地址
movl 12(%ebp), %edx //x
movl %edx, (%eax) //p->x=x
movl 8(%ebp), %eax
movl 16(%ebp), %edx //y
movl %edx, 4(%eax) //p->y=y
popl %ebp
ret
main:
pushl %ebp
movl %esp, %ebp
subl $28, %esp
movl $10, -8(%ebp) //x=10
movl $20, -4(%ebp) y=20
movl -4(%ebp), %eax
movl %eax, 8(%esp)
movl -8(%ebp), %eax
movl %eax, 4(%esp)
leal -16(%ebp), %eax //取p地址&p
movl %eax, (%esp)
call PointInit
movl $0, %eax
leave
ret
080483b4 <ArraySum>:
80483b4: 55 push %ebp
80483b5: 89 e5 mov %esp,%ebp
80483b7: 83 ec 10 sub $0x10,%esp
80483ba: c7 45 f8 00 00 00 00 movl $0x0,-0x8(%ebp)
80483c1: c7 45 fc 00 00 00 00 movl $0x0,-0x4(%ebp)
80483c8: eb 12 jmp 80483dc <ArraySum+0x28>
80483ca: 8b 45 fc mov -0x4(%ebp),%eax
80483cd: c1 e0 02 shl $0x2,%eax
80483d0: 03 45 08 add 0x8(%ebp),%eax
80483d3: 8b 00 mov (%eax),%eax
80483d5: 01 45 f8 add %eax,-0x8(%ebp)
80483d8: 83 45 fc 01 addl $0x1,-0x4(%ebp)
80483dc: 8b 45 fc mov -0x4(%ebp),%eax
80483df: 3b 45 0c cmp 0xc(%ebp),%eax
80483e2: 7c e6 jl 80483ca <ArraySum+0x16>
80483e4: 8b 45 f8 mov -0x8(%ebp),%eax
80483e7: c9 leave
c3 ret :
作者:coderkian
出处:http://www.cnblogs.com/coderkian/
本文版权归作者和博客园共有,欢迎转载,但未经作者同意必须保留此段声明,且在文章页面明显位置给出原文连接,否则保留追究法律责任的权利。
原文始发于微信公众号(汇编语言):linux下汇编语言开发总结
免责声明:文章中涉及的程序(方法)可能带有攻击性,仅供安全研究与教学之用,读者将其信息做其他用途,由读者承担全部法律及连带责任,本站不承担任何法律及连带责任;如有问题可邮件联系(建议使用企业邮箱或有效邮箱,避免邮件被拦截,联系方式见首页),望知悉。
- 左青龙
- 微信扫一扫
-
- 右白虎
- 微信扫一扫
-
评论